Exploring Tokens in JavaScript (2024 and Beyond)
- amuggs82
- Dec 9, 2024
- 5 min read
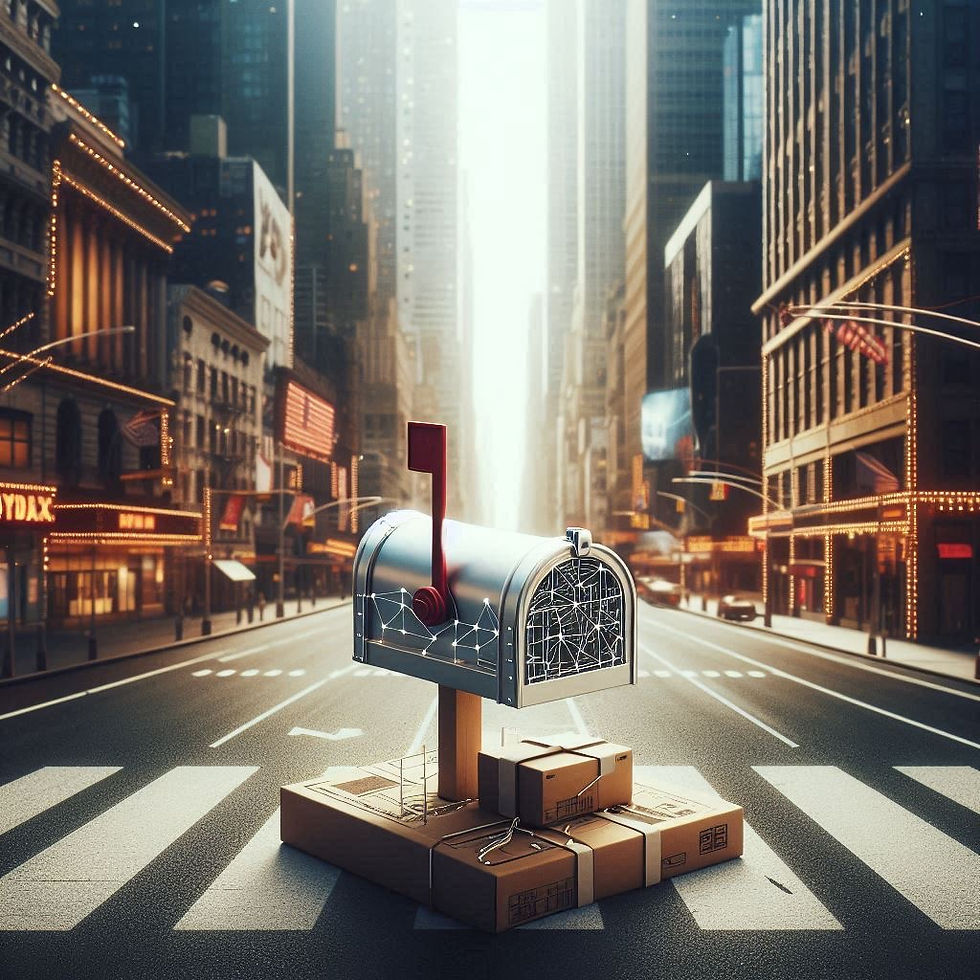
Overview of Tokens
Tokens are vital in modern software development, particularly in authentication and authorisation for APIs, microservices, and distributed systems. Tokens serve as compact, self-contained data structures that encode claims and attributes about an entity (usually a user or service).
In 2024, JSON Web Tokens (JWTs) remain a widely used standard for secure communication between systems.
Design Factors of Tokens
1. Structure of JWT
A JWT consists of three components:
Header: Specifies metadata, including the algorithm used for signing (e.g., HS256 or RS256).
Payload: Encodes claims about the entity (e.g., user ID, roles, expiration time).
Signature: Ensures token integrity by signing the base64-encoded header and payload using a secret or public/private key pair.
Example of a JWT:
{
"header": {
"alg": "HS256",
"typ": "JWT"
},
"payload": {
"sub": "1234567890",
"name": "Tarah Querfurt",
"iat": 1696843471
},
"signature": "signature_hash"
}
2. Design Considerations
Compactness: Tokens should be small to optimise transmission over networks.
Statelessness: Tokens are self-contained, reducing the need for server-side storage.
Extensibility: Claims can be customised to meet specific business requirements.
Expiration: Tokens include exp claims to enforce limited validity.
3. Encapsulation
JWTs are not inherently encapsulated but can be encapsulated by encrypting the token (JWE) to ensure confidentiality alongside integrity. Tools like libraries in Node.js (jsonwebtoken) allow developers to handle both signed (JWS) and encrypted tokens.
Benefits of JWTs
Scalability: Statelessness makes them ideal for distributed systems, eliminating the need for session persistence.
Interoperability: Being based on JSON and supported by many libraries, they integrate seamlessly across languages and platforms.
Performance: Tokens allow quick validation (via signature) without querying a database.
Drawbacks of JWTs
Size: JWTs can become large if overloaded with claims.
Revocation: Stateless tokens are difficult to revoke; systems must rely on token expiration or maintain a revocation list.
Exposure Risks: If leaked, JWTs can be misused unless additional safeguards, such as short expiration times or refresh tokens, are applied.
Security and Interaction in Microservices
1. Interaction with Microservices
Tokens are passed in HTTP headers (e.g., Authorisation: Bearer <token>).
Microservices validate tokens using the issuer’s public key (if signed using asymmetric encryption like RS256).
Access control is enforced by inspecting claims (e.g., roles or scopes).
2. Ensuring Security
Encryption: Use JWE for sensitive claims.
Short TTL: Limit token lifetime to reduce exposure risks.
Refresh Tokens: Issue long-lived refresh tokens alongside short-lived JWTs.
Validation: Use a library like jsonwebtoken to validate the token’s signature and claims.
Rate Limiting: Prevent brute-force attacks against token endpoints.
Tokens in Object-Oriented Programming (OOP)
1. Are Tokens Objects?
Tokens can be modeled as objects in OOP. For instance:
class Token {
constructor(header, payload, signature) {
this.header = header;
this.payload = payload;
this.signature = signature;
}
isExpired() {
return Date.now() / 1000 > this.payload.exp;
}
}
2. Immutability
Tokens are designed to be immutable. Once issued, their claims cannot be altered without invalidating the signature. This immutability ensures integrity.
Future Standards (2025 and Beyond)
While JWTs will likely remain dominant, emerging standards may address current limitations:
OAuth 2.1: Consolidates OAuth practices, reducing complexity and vulnerabilities.
JSON Object Signing and Encryption (JOSE): May extend to provide better mechanisms for token confidentiality.
Passkeys: Tokens could integrate with FIDO2/WebAuthn to provide seamless, password-less authentication.
Conclusion
Tokens, especially JWTs, are indispensable in secure communication and microservices architecture. Their compact design, self-containment, and ease of use have cemented their place as a standard. With advancements in cryptography and protocol design, the tokenization landscape will continue to evolve to address scalability, revocation, and enhanced privacy concerns.
Technical Terms
1. JSON (JavaScript Object Notation)
A lightweight data-interchange format that is easy to read and write for humans and easy to parse and generate for machines.
Example: { "key": "value" }
MReference: RFC 7159, JSON Data Interchange Format.
2. Statelessness
A design principle in which each request from a client to a server must contain all the information needed to understand and process it, without relying on stored context on the server.
Example: REST APIs are typically stateless.
3. Encapsulation
The concept of restricting direct access to some of an object’s components in OOP to ensure data integrity.
In token security, encapsulation involves encrypting tokens to prevent unauthorised access.
4. Claims
Pieces of information asserted by the token about the entity (e.g., a user).
Example: A JWT might contain a claim { "role": "admin" } indicating the user’s role.
5. TTL (Time-to-Live)
A mechanism that defines the lifespan of data, such as the expiration of a token. Tokens often include a exp (expiration) claim to enforce this.
6. Integrity
Assurance that data has not been altered during transmission. Token integrity is maintained through cryptographic signatures.
7. Mutability/Immutability
Mutable: Data that can be changed after creation.
Immutable: Data that cannot be changed once created. Tokens are typically immutable to ensure integrity.
8. Cryptography
The practice and study of securing communications through techniques like encryption and hashing. Cryptography underpins token security.
Reference: Stallings, W. Cryptography and Network Security.
9. Authorisation
The process of determining if a user or service has permission to access a resource. Tokens often carry authorisation information.
10. Distributed Systems
A collection of independent computers that appear to the user as a single coherent system. Tokens facilitate secure communication in such systems.
Acronyms
1. JWT (JSON Web Token)
A compact, URL-safe means of representing claims to be transferred between two parties.
Composed of three parts: Header, Payload, and Signature.
Reference: RFC 7519, JSON Web Token (JWT).
2. JWS (JSON Web Signature)
A JSON-based standard for creating signatures on data, commonly used in JWTs.
Reference: RFC 7515, JSON Web Signature (JWS).
3. JWE (JSON Web Encryption)
A standard for encrypting data to protect its confidentiality.
Reference: RFC 7516, JSON Web Encryption (JWE).
4. OOP (Object-Oriented Programming)
A programming paradigm based on the concept of “objects,” which are instances of classes encapsulating data and behaviour.
Reference: Gamma, E., et al. Design Patterns: Elements of Reusable Object-Oriented Software.
5. REST (Representational State Transfer)
An architectural style for designing networked applications that uses stateless communication, often with HTTP.
6. TTL (Time-to-Live)
A limit on the lifespan or validity of data, ensuring it is automatically discarded after a specific time.
7. RSA (Rivest–Shamir–Adleman)
A widely used public-key cryptographic algorithm.
Example: Used for asymmetric encryption in token signing (e.g., RS256).
8. HMAC (Hash-based Message Authentication Code)
A cryptographic method combining a secret key and a hash function to ensure data integrity and authenticity.
Example: HS256 in JWTs.
9. OFDMA (Orthogonal Frequency Division Multiple Access)
A multi-user version of OFDM, allowing multiple users to share a channel in time and frequency domains. Though not directly related to JWT, it’s relevant in distributed systems communication.
References for Further Reading
1. RFC 7519: JSON Web Token (JWT
References for Further Reading:
1. RFC 7519: JSON Web Token (JWT).
2. Bösch, C., et al. “Cryptographic Protocols for Privacy.” ACM Computing Surveys.
3. BSI. “Secure Token Guidelines for Distributed Systems.”
Comments